Array
Why do we need an array?
Let’s consider the situation where we need to get 10 student’s age and store it for some calculation.
Since age is an integer type, we can store it something like below,
Example
int ag1, age2, age3, age4, age5, age6, age7, age8, age9, age10;
if we declare like above, it will be very difficult for us to manipulate the data.
If more number of student joins, then it is very difficult to declare a lot of variables and keep track of it.
To overcome this kind of situation, we should use Array data structure.
What is an Array?
An array is a collection of variables in same datatype.
we can’t group different data types in array.
Like, combination of integer and char, char and float etc.
Hence Array is called as homogeneous data type.
Example
If we consider house as a datatype then apartment is an array of house.
Pictorial Explanation
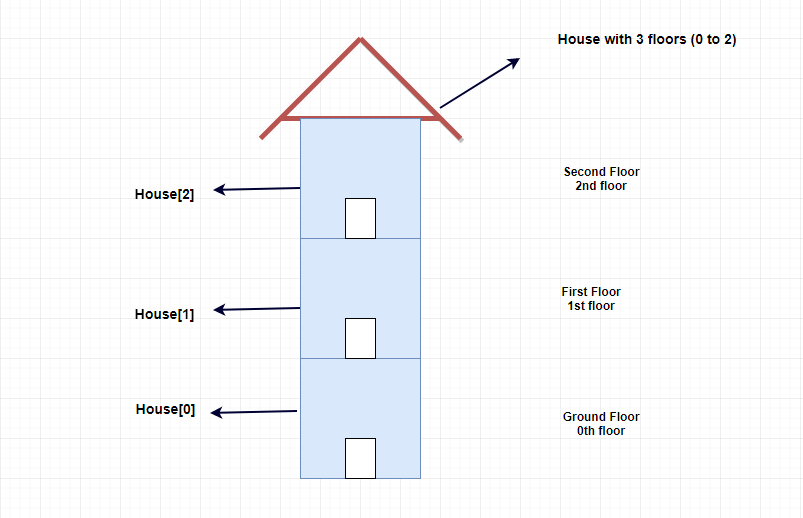
The above apartment has 3 house. i.e
House[0] -> first house
House[1] -> second house
House[2] ->third house.
Likewise, we can use array to solve our problem.
Use Array to store ages
To store 10 students age, we can simply declare array like below,
Example
int age[10];
Array index starts from 0 not 1.
To access 1st student’s age, we can directly use index 0. i.e age[0]
To access 5th student’s age, we can directly use index 4. i.e age[4]
We can manipulate Nth students age by using index N-1. Where N > 0
In general, an array of size N will have elements from index 0 to N-1.
Using index, we can directly access desired element.
Pictorial Explanation
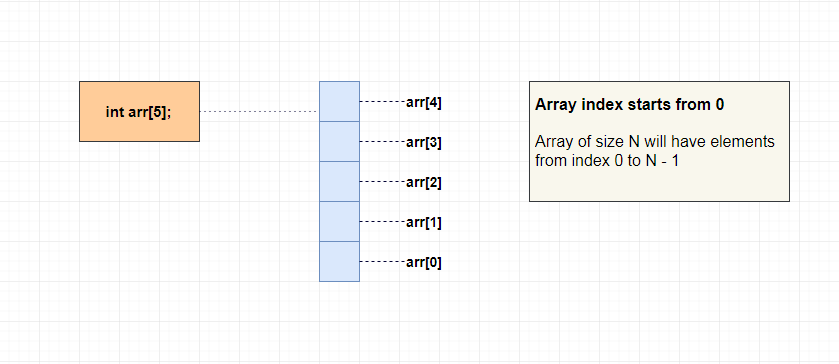