Declaring an Array
To declare an array,
1.We should specify the datatype of the array
2.Name of the array
3.And required size with in square brackets.
Syntax
To declare a single dimensional array,
Example
datatype array_name[size];
Where,
Datatype can be int, float, double, char etc.
array_name should be a valid variable name.
size is an integer value.
size should not be a negative value.
Example
Example
int age[10]; char name[100]; float weight[5];
Initialization of an Array
We can initialize an array while declaring it. Like below,
Example
int arr[5]={10,20,30,40,50};
Pictorial Explanation
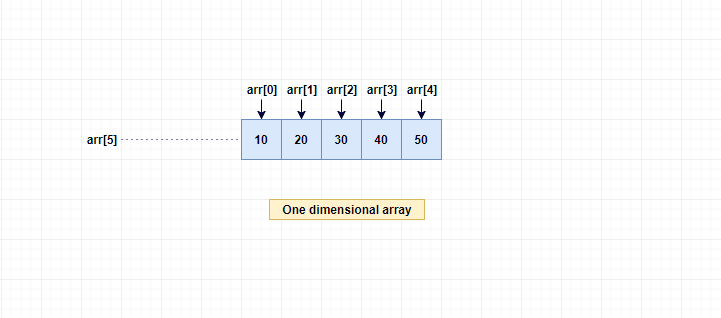
We can also let the compiler to determine the array size by not giving the initial array size while initializing it.
In this case, array size will be determined by compiler.
Example
int arr[]={10,20,30,40,50};
Getting input from user
we can get input from the user and store it in array using loop.
In the case of array, we know exact array size while initializing it, so we should use for loop.
Example
#include<stdio.h> int main() { int arr[5]; //array of lengeth 5 type integer.. arr[0]-->arr[4] int i; for(i = 0; i < 5; i++) //for getting 5 elements from user scanf("%d",&arr[i]); for(i = 0; i < 5; i++) //printing all 5 elements printf("%d\n",arr[i]); return 0; }
It will get 5 elements from the user and print it.