Switch Statement
Switch statement allows us to pick one options at a time among many options.
If none of them are match, then default case will execute.
Example
Assume that we are going to program a simple calculator where user can select the operation by giving the choice.
Say,
If they press 1, we should show the sum.
If they press 2, we should show the subtraction.
And so on.
These kind of situation, we should go for switch statement.
Syntax
Example
switch(choice) { case 1: //statement break; case 2: //statement break; default: //statement }
Choice should be an integer or a character datatype.
Other datatypes are not allowed. i.e. float,double,string
Sample Program
Example
#include<stdio.h> int main() { int num1, num2, choice; printf("Enter two numbers\n"); scanf("%d%d",&num1,&num2); printf("Press \n 1 for sum \n 2 for sub \n 3 for mul \n 4 for div\n"); scanf("%d",&choice); switch(choice) { case 1: printf("Sum = %d\n",num1 + num2); break; case 2: printf("Sub = %d\n",num1 - num2); break; case 3: printf("Mul = %d\n",num1 * num2); break; case 4: printf("Div = %d\n",num1 / num2); break; default: printf("Enter valid choice\n"); } return 0; }
Simple calculator program with user choice.
Why break statement ?
break statement is used to terminate switch statement execution, once we have achieved our result.
For example, if case 1 is our choice, then no need to check for case 2, 3 and so on.
What will happen if we don’t give the break statement inside switch?
Let’s discuss it with the following program.
Consider the same program without break.
Example
#include<stdio.h> int main() { int num1, num2, choice; printf("Enter two numbers\n"); scanf("%d%d",&num1,&num2); printf("Press \n 1 for sum \n 2 for sub \n 3 for mul \n 4 for div\n"); scanf("%d",&choice); switch(choice) { case 1: printf("Sum = %d\n",num1 + num2); case 2: printf("Sub = %d\n",num1 - num2); case 3: printf("Mul = %d\n",num1 * num2); case 4: printf("Div = %d\n",num1 / num2); default: printf("Enter valid choice\n"); } return 0; }
What will be the output if we enter the choice as 1?
Here, we did not give the break statement. So, it will print every case result.
Like below,
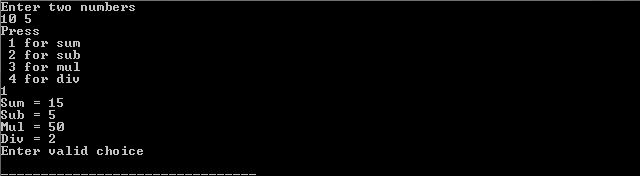
What will be the output if we enter choice as 3?
It will print results from case 3 to end.
So, output will be
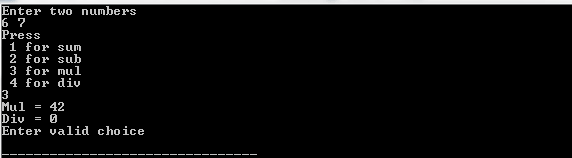