return statement
When program execution reaches the return statement, it will leave the current function and the program execution will resume to the next statement, where it was actually called.
Syntax
Example
return;
or
Example
return (value or variable); return 10; return a; return a+b;
The value will be passed back to the function where it was called. We can receive the value and do the further programming from that point.
Pictorial Explanation
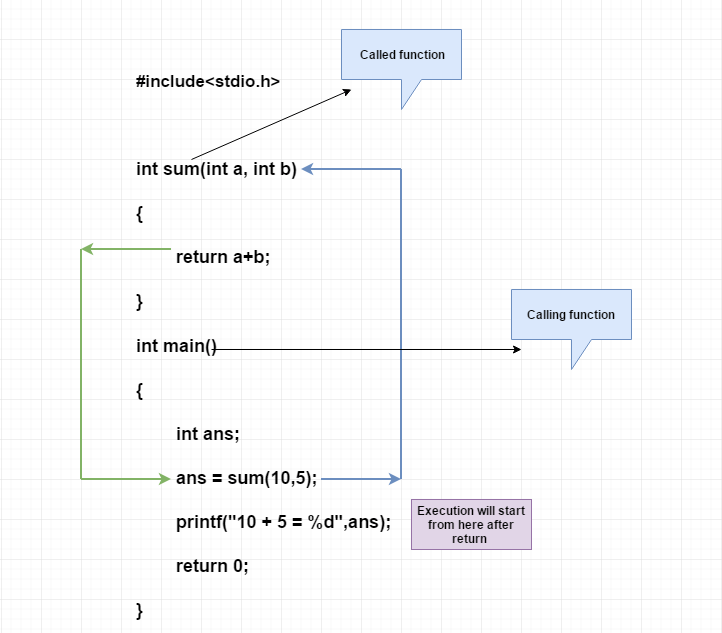
Where,
Calling function - Main function (the point from where the function is called)
Called function - the actual function which going to execute.
ans will receive the sum result that is 15. in other words, ans will hold value 15 after the execution of return statement.
And the program execution will resume from printf("10 + 5 = %d",ans); statement.
What will happen if we write some statements below to the return statement?
If the program execution reaches return statement, the statements below to that will not be executed.
Execution control will leave the current function immediately.
Example
#include<stdio.h> int sum(int a, int b) { return a+b; /* * printf will not be executed, * as it is below to the return statement */ printf("Hello"); } int main() { int ans; ans = sum(10,5); //ans will have the return value i.e a+b printf("10 + 5 = %d",ans); return 0; }
In the above program, printf("Hello"); will not be executed as it's below to the return statement.