break statement
Break statement is used to terminate the loop and resume the program execution from the next statement following the loop.
If we want to use break statement inside loop, we have to use it along with if statement.
It is also used to terminate the switch case execution.
Example
Get integer input from user and print it continuously until the user gives a negative number.
Program
Example
#include<stdio.h> int main() { int num; while(1) //It is known as infinite loop since the condition always 1 { scanf("%d",&num); //getting input from user if(num < 0) // if num is less than zero break; //terminate the loop printf("%d\n",num); //Printing the number } return 0; }
If user gives a negative number, the loop will terminate and the statement next to loop will execute which is return 0.
Pictorial Explanation
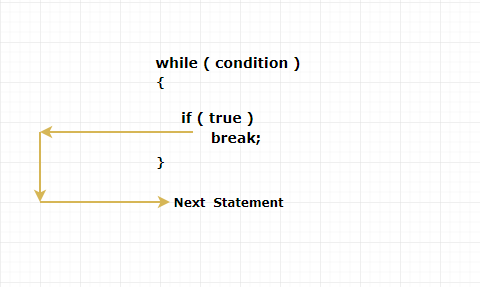