continue statement
Continue statement is used in looping to skip some statements.
It is used inside loop along with the decision-making statements.
When continue statement encounters, the execution will go to the starting of the loop.
The statements below continue statement will not be executed, if the given condition becomes true.
Example
Print number continuously from 1 to 100 except 25,50 and 75.
In this program, we should print numbers from 1 to 100 using for loop but when the number is equals to 25 or 50 or 75, we should give continue statement.
Program
Example
#include<stdio.h> int main() { int i; for(i=1;i<=100;i++) { if(i == 25 || i == 50 || i == 75) continue; printf("%d\n",i); } return 0; }
If we run the above program, it will print numbers from 1 to 100 except the numbers 25,50 and 75.
Pictorial Explanation
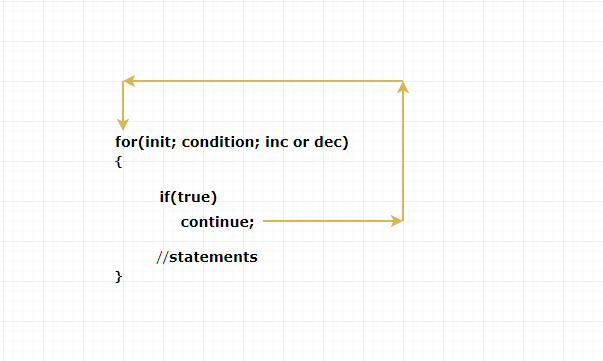