while loop
When we don’t know the exact iteration count or the loop count, we should use while loop.
In other words, if iteration count depends on the input, we should use while loop
How many times a given number can be divided by 2 till it becomes 1.
If the user gives input as 2
Then loop will execute 1 time. i.e 2/2 = 1
If the user gives input as 8
Then loop will execute 3 times. i.e 8/2 = 4. 4/2 = 2. 2/2 = 1.
Here iteration count is depending on the input. For loop will not suit here because we don’t know the exact iteration count.
Syntax
Example
initialize variable while(condition) { //statements in(de)crement variable }
Statements inside while loop will repeat til the condition becomes true.
1.It will check the condition
2.if the condition is true
3.Statements will execute.
4.And then increment or decrement the value of variable
5.Again, it will go to step 2
6.else
7.Execution will come out of while loop
Sample program
Get an integer input from user and print how many times it can be divided by 2 till it becomes 1.
Example
Case 1
Input
8
Output
3 ( 8/2 = 4 -> 4/2=2 -> 2/2=1)
Case 2
Input
20
Output
4(20/2=10 -> 10/2=5 -> 5/2=2 -> 2/2=1)
Example
#include<stdio.h> int main() { int num,count = 0; scanf("%d",&num); while(num > 1) { count++; //increment count variable num = num >> 1; //equal to num = num / 2. } printf("%d",count); return 0; }
Pictorial Explanation
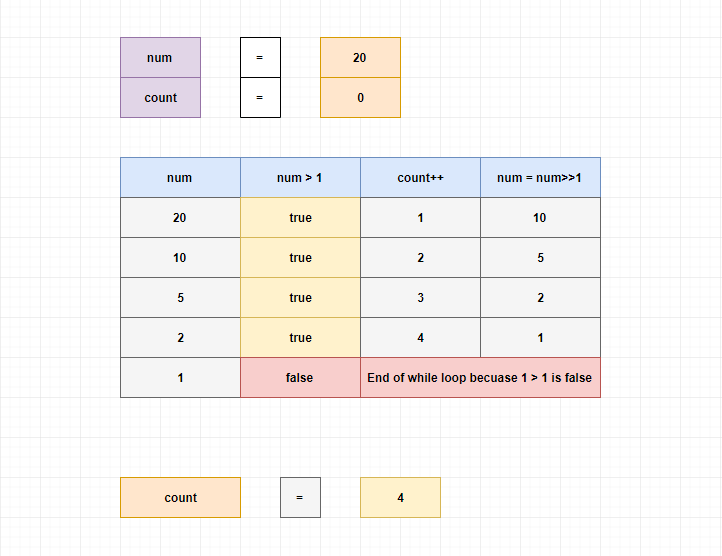