Assignment Operator in C
Using assignment operators, we can assign value to the variables.
Equality sign (=) is used as an assignment operator in C.
Example
int var = 5;
Here, value 5 has assigned to the variable var.
Example
#include<stdio.h> int main() { int a = 10; int b = a; printf("a = %d \t b = %d\n",a,b); return 0; }
Here, value of a has assigned to the variable b. Now, both a and b will hold value 10.
Basically, the value of right-side operand will be assigned to the left side operand.
Pictorial Explanation
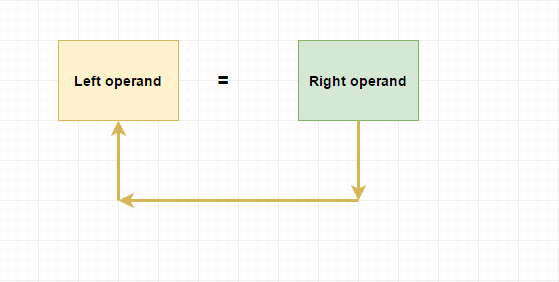
Compound assignment operators
Operator |
Meaning |
Example |
---|---|---|
+= |
L=L+R |
a+=b;same as a=a+b |
-= |
L=L-R |
a-=b;same as a=a-b |
*= |
L=L*R |
a*=b;same as a=a*b |
/= |
L=L/R |
a/=b;same as a=a/b |
%= |
L=L%R |
a%=b;same as a=a%b |
Sample Program
Example
#include<stdio.h> int main() { int a = 10, b = 5; a+=b; // same as a=a+b printf("value of a+b = %d\n",a); // a will hold the result return 0; }