2D array and pointers
In this tutorial, we are going to learn the relationship between 2d array and pointers.
2D array
Let's declare a 3x3 array.
int arr[3][3];
Let's assume the starting address of the above 2D array as 1000.
Visual Representation
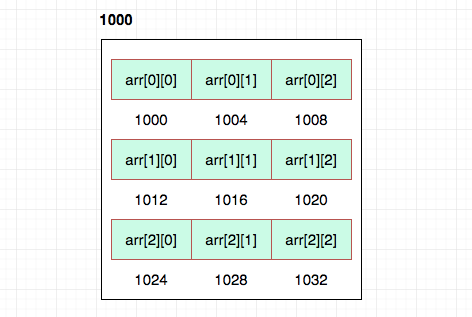
The above array's memory address is an arithmetic progression with common difference of 4 as the size of an integer is 4.
&arr is a whole 2D array pointer
&arr is a pointer to the entire 2D(3x3) array. i.e. (int*)[3][3]
If we move &arr by 1 position(&arr+1), it will point to the next 2D block(3X3).
In our case, the base address of the 2D array is 1000. So, &arr value will be 1000.
What will be the value of &arr+1?
In general, for Row x Col array the formula will be base address + (Row * Col) * sizeof(array datatype)
In our case, Row = 3, Col = 3, sizeof(int) = 4.
It is base address + (3 * 3)* 4 => 1000 + 36 => 1036
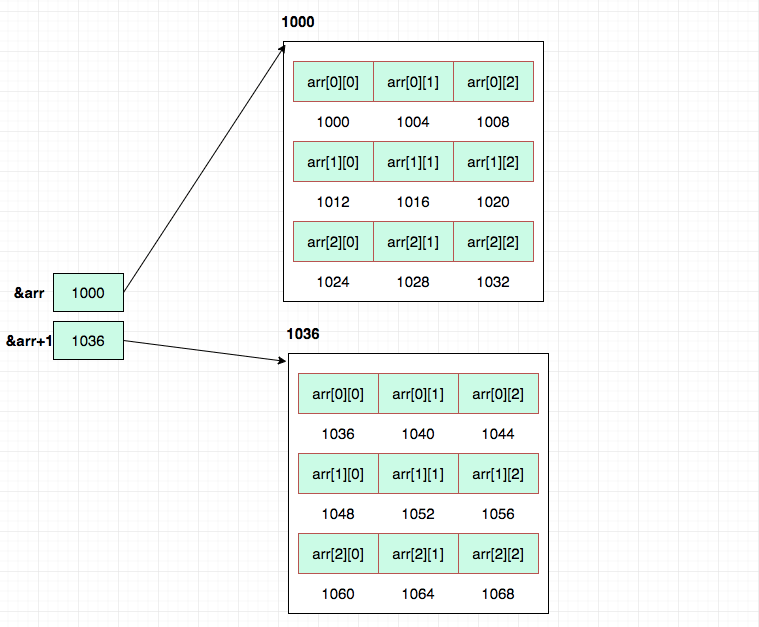
arr is a 1D array pointer
arr is a pointer to the first 1D array. i.e. (int*)[3]
If we move arr by 1 position(arr+1), it will point to the next 1D block(3 elements).
The base address of first 1D array also 1000. So, arr value will be 1000.
What will be the value of arr+1?
It will be base address + Row * sizeof(datatype).
1000 + 3 * 4
1012
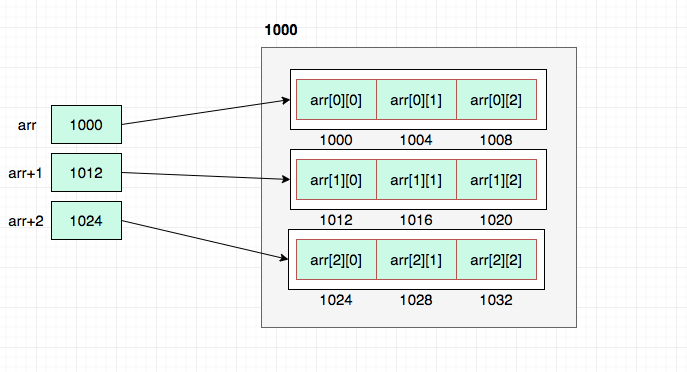
*arr is a pointer to the first element of the 2D array.
*arr is a pointer to the first element of the 2D array. i.e. (int*)
If we move *arr by 1 position(*arr+1), it will point to the next element.
The base address of the first element also 1000.So, *arr value will be 1000.
What will be the value of *arr+1?
It will be base address + sizeof(datatype).
1000 + 4
1004
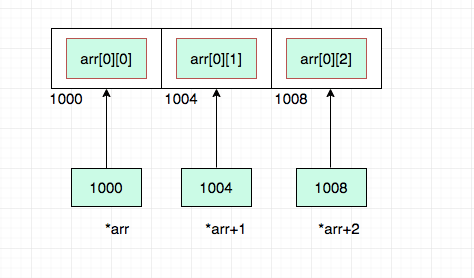
**arr will be the value of the first element.
Since *arr holds the address of the first element, **arr will give the value stored in the first element.
If we move **arr by 1 position(**arr+1), the value will be incremented by 1.
If the array first element is 10, **arr+1 will be 11.
Summary
1. &arr is a 2D array pointer (int*)[row][col]. So, &arr+1 will point the next 2D block.
2. arr is a 1D array pointer (int*)[row]. So, arr+1 will point the next 1D array in the 2D array.
3. *arr is a single element pointer (int*). So, *arr+1 will point the next element in the array.
4. **arr is the value of the first element. **arr+1 will increment the element value by 1.