Accessing array elements using pointers
we can access the array elements using pointers.
Example
int arr[5] = {100, 200, 300, 400, 500}; int *ptr = arr;
Where
ptr is an integer pointer which holds the address of the first element. i.e &arr[0]
ptr + 1 points the address of second variable. i.e &arr[1]
Similarly, ptr + 2 holds the address of the third element of the array. i.e. &arr[2]
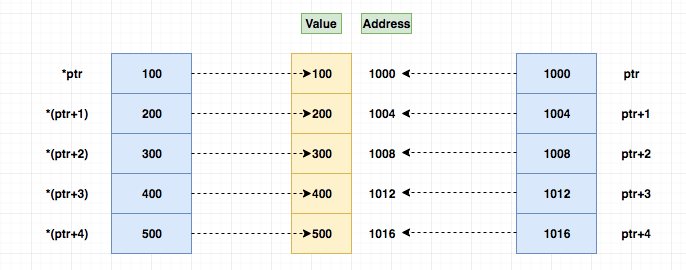
Printing each array elements address and value
Example
#include<stdio.h> int main() { int arr[5] = {100, 200, 300, 400, 500}, i; int *ptr = arr; //Address ptr+i //Value stored at the address *(ptr+i) for(i = 0; i < 5; i++) printf("&arr[%d] = %p\t arr[%d] = %d\n",i,ptr+i,i,*(ptr+i)); return 0; }
Modifying array elements using the pointer
Let's change the 3rd (index 2) element as 1000.
Example
#include<stdio.h> int main() { int arr[5] = {100, 200, 300, 400, 500}, i; int *ptr = arr; //changing 3rd element(300) as 1000. *(ptr+2) = 1000; for(i = 0; i < 5; i++) printf("arr[%d] = %d\n",i,*(ptr+i)); return 0; }