Address of a variable
What will happen in the computer memory, if we declare and assign a value to a variable?
int var = 10;
It will allocate 4 bytes in the memory as it is an integer variable.
Each memory location will have unique memory address .
variable var will be mapped to particular memory address.
And it will store value 10 at this memory address.
Like below,
Pictorial Explanation
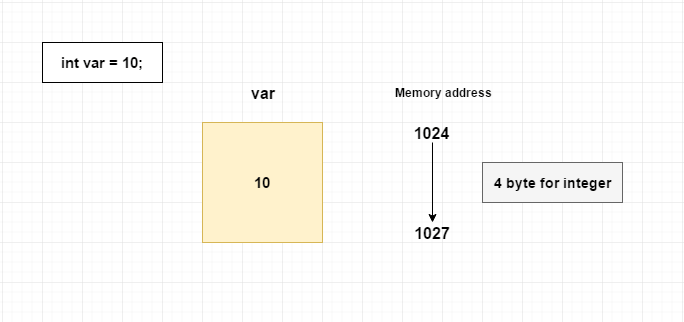
Let us assume starting memory address as 1024.
Then the variable var will be mapped to memory address 1024 and it will reserve 4 bytes starting from 1024 to 1027.
How to print the address of a variable?
We can print the address of a variable using & operator. i.e address of operator
Example
#include<stdio.h> int main() { int var = 10; /*using & operator, we can print the address of a variable */ printf("%x",&var); return 0; }
%x format specifier in printf used to print the address in hexadecimal format.
Let's assume output as 1024 (address of var).
Now, can you guess the output of the below program?
It's not 1025! It's 1028 since var is an integer variable it will reserve 4 bytes to store the value.
So, if we move the integer variable address by 1, it will move 4 bytes i.e. 1024 + 4 = 1028.
Likewise, if we move character variable address by 1, it will move 1 byte as character variable reserve 1 byte to store the value.
In general, if we add n to particular data type's variable address,
the output will be = base address + (n * sizeof(data type))
Example
Assume &var as 1024
then &var+5 will be,
= 1024 + 5 * sizeof(int)
= 1024 + 5 * 4
= 1024 + 20
= 1044