Array and Pointers
An array is a collection of variables of the same datatype.
In C language, array and pointers are more or less same.
Example
int arr[5]={10,20,30,40,50};
where,
arr will point the first element in the array which is 10.
Hence, arr will have the address of arr[0] (&arr[0]).
arr is exactly as same as &arr[0]. arr == &arr[0]
arr+i
Assume that the first element address as 1024. So, arr will point the memory address 1024.
What will happen if we move arr by 1 position? i.e. arr+1?
It will not add 1 to 1024 instead it will move 4 bytes of memory as the size of an integer is 4 bytes.
So, arr + 1 will be 1028.
arr + 1 will point the second element of an array.
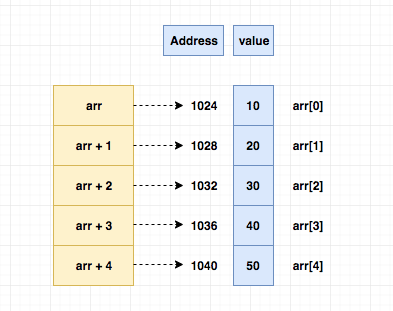
Printing array elements address
Example
#include<stdio.h> int main() { int arr[5] = {10, 20, 30, 40, 50}, i; for(i = 0; i < 5; i++) printf("Address of arr[%d] = %p\n",i,arr+i); return 0; }
%p format specifier is used to printing the pointer address.
arr[i] == *(arr+i)
arr[i] is exactly same as *(arr+i) (value stored at the address arr+i).
arr[i] will give the value stored at the memory address arr + i.
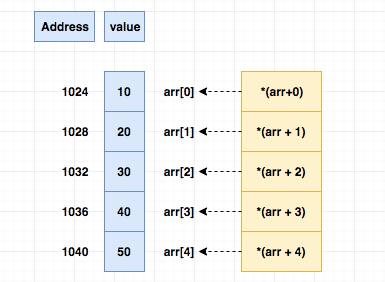
Printing elements in the array
Example
#include<stdio.h> int main() { int arr[5] = {10, 20, 30, 40, 50}, i; for(i = 0; i < 5; i++) printf("value stored in arr[%d] = %d\n",i,*(arr+i)); return 0; }