Array of pointers in c
Like an array of variables, we can also use array of pointers in c.
In this tutorial explains the array of pointers and its application.
Array of pointer
Let's create an array of 5 pointers.
int *arr[5];
Where arr[0] will hold one integer variable address, arr[1] will hold another integer variable address and so on.
Example
/* * Program : Array of Pointers * Language : C */ #include<stdio.h> #define size 5 int main() { int *arr[size]; int a = 10, b = 20, c = 30, d = 40, e = 50, i; arr[0] = &a; arr[1] = &b; arr[2] = &c; arr[3] = &d; arr[4] = &e; printf("Address of a = %p\n",arr[0]); printf("Address of b = %p\n",arr[1]); printf("Address of c = %p\n",arr[2]); printf("Address of d = %p\n",arr[3]); printf("Address of e = %p\n",arr[4]); for(i = 0; i < size; i++) printf("value stored at arr[%d] = %d\n",i,*arr[i]); return 0; }
Dereferencing array of pointer
Dereference - Accessing the value stored at the pointer.
Since each array index pointing to a variable's address, we need to use *arr[index] to access the value stored at the particular index's address.
arr[index] will have address
*arr[index] will print the value.
Example
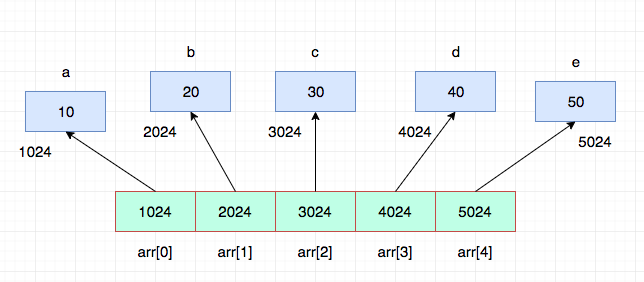
Where arr[0] holding the address of the variable a. i.e. arr[0] = 1024
*arr[0] -> *1024 -> value stored at the memory address 1024 is 10. So, *arr[0] = 10.
Similarly, *arr[1] = 20, *arr[2] = 30 and so on.
Application of array of pointers
Assume that we are going to build an embedded system which uses a different kind of sensors to measure the temperature.
In this case, we can use an array of pointers to hold the memory address of each sensor so that it will be very easy to manipulate the sensor status.
Example
sensor[0] will hold the address of the 1st sensor.
sensor[1] will hold the address of the second sensor and so on.
Since it is an array, we can directly interact with the particular sensor using array index.
we can get the temperature status of 1st sensor using sensor[0], 2nd sensor status using sensor[1] and so on.