Call by value and call by reference
In this tutorial, we will discuss the difference between call by value and call by reference with an example.
Animated Tutorial
Call by value
If we call a function with value (variable name), the value will be duplicated and the function will receive it.
#include<stdio.h> void print(int a) { printf("From print function ...\n"); printf("Address of a = %p\n",&a); } int main() { int a = 10; printf("From Main Function ...\n"); printf("Address of a = %p\n",&a); print(a); return 0; }
Output
From Main Function ...
Address of a = 0x7ffee9fcfbd8
From print function ...
Address of a = 0x7ffee9fcfbbc
We can observe that the address of a in the main function and the address of a in print function are different.
So, if we change the value of a in print function, it will not affect the value of a in the main function.
/* * Program : Call by value example * Language : C */ #include<stdio.h> //set the argument value to 0 void set(int a) { a = 0; printf("Set : In set function a = %d\n",a); } int main() { int a = 10; printf("Main : Before calling set function a = %d\n",a); set(a); printf("Main : After calling set function a = %d\n",a); return 0; }
Output
Main : Before calling set function a = 10
Set : In set function a = 0
Main : After calling set function a = 10
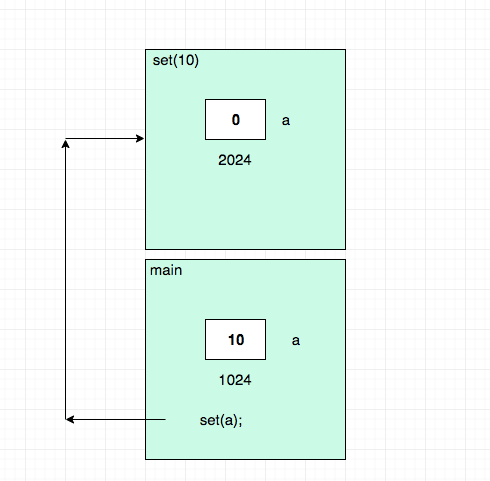
Call by reference
In call by reference, we pass the address of a variable.
So, if we modify the value, it will affect the original value of a variable.
#include<stdio.h> void print(int *a) { printf("From print function ...\n"); printf("Address of a = %p\n",a); } int main() { int a = 10; printf("From Main Function ...\n"); printf("Address of a = %p\n",&a); print(&a); return 0; }
From Main Function ...
Address of a = 0x7ffee2ea4bd8
From print function ...
Address of a = 0x7ffee2ea4bd8
We can observe that the address of a in the main function and the address of a in print function are same.
/* * Program : Call by reference example * Language : C */ #include<stdio.h> //set the argument value to 0 void set(int *a) { *a = 0; printf("Set : In set function a = %d\n",*a); } int main() { int a = 10; printf("Main : Before calling set function a = %d\n",a); set(&a); printf("Main : After calling set function a = %d\n",a); return 0; }
Output
Main : Before calling set function a = 10
Set : In set function a = 0
Main : After calling set function a = 0
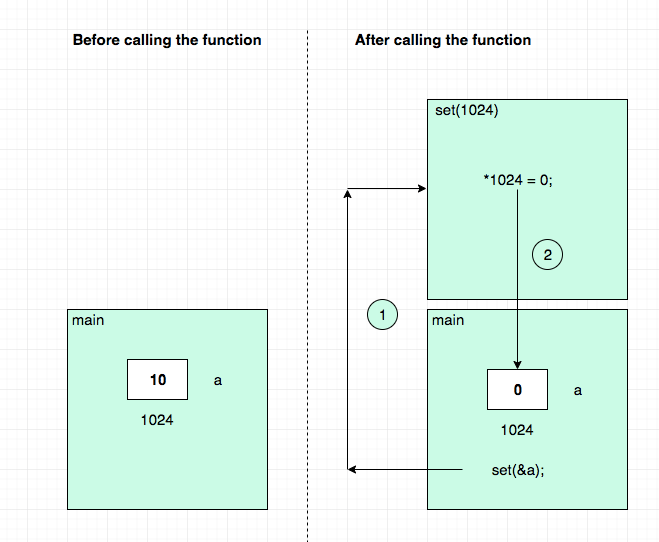