free
It's mandatory to deallocate the memory area which has created dynamically when the memory is no longer needed.
Why do we need to deallocate the dynamic memory?
When we try to create the dynamic memory, the memory will be created in the heap section.
Memory leak
If we don't deallocate the dynamic memory, it will reside in the heap section.It is also called memory leak.
It will reduce the system performance by reducing the amount of available memory.
Let's assume total heap size as K.
If we allocate N byte of memory dynamically, it will consume N bytes of memory in heap section.
When the particular piece of code executed M number of time, then M * N bytes of memory will be consumed by our program.
At some point in time (M * N > K), the whole heap memory will be consumed by the program it will result in the system crash due to low available memory.
Pictorial Explanation
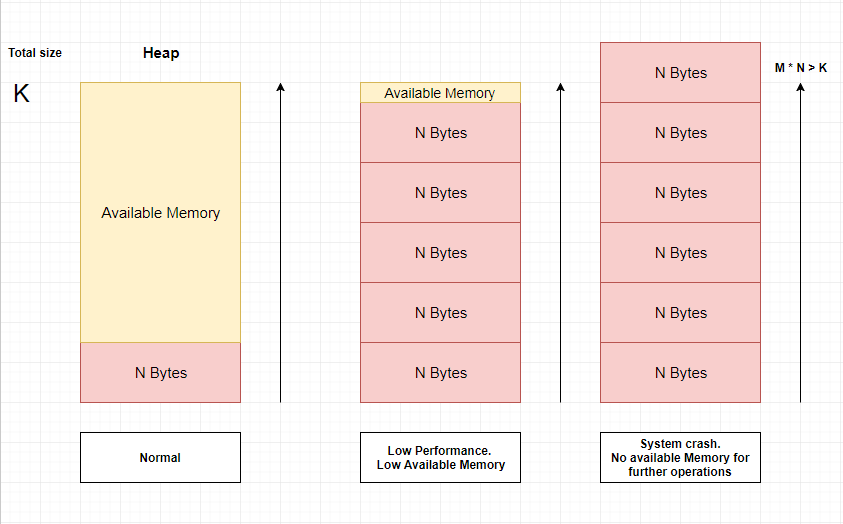
So, it is programmers responsibility to deallocate the dynamic memory which is no longer needed.
Animated Tutorial
How to deallocate the dynamic memory?
using free() function, we can deallocate the dynamic memory.
Syntax of free
free(ptr);
Example
char *ptr; ptr = malloc(N); //do something free(ptr);
Pictorial Explanation
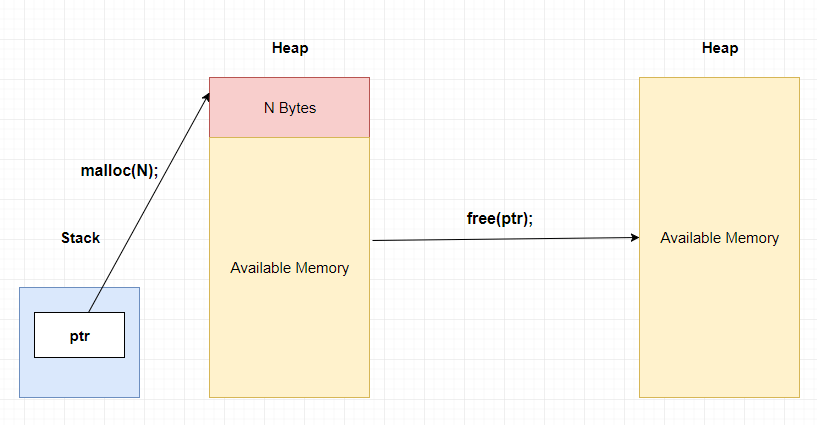
Sample program
Sum of N numbers. Get N from the user and use dynamic memory to store the inputs.
Example
#include<stdio.h> #include<stdlib.h> int main() { int *ptr,n,i,sum = 0; //get number of elements scanf("%d",&n); //allocate dynamic memory ptr = malloc(n * sizeof(int)); //if success if(ptr != NULL) { //get input from the user for(i = 0; i < n; i++) scanf("%d", ptr + i); //add all elements for(i = 0; i < n; i++) sum += *(ptr + i); //print the result printf("sum = %d\n",sum); //deallocate the memory free(ptr); } return 0; }
Double free is undefined
If we free the same pointer two or more time, then the behavior is undefined.
So, if we free the same pointer which is freed already, the program will stop its execution.
Example
char *ptr; ptr = malloc(10); free(ptr); free(ptr);