Integer pointer arithmetic
What will be the expected output of the below program?
Example
#include<stdio.h> int main() { int a = 10, *ptr; //assume base address of variable 'a' as 1024 //ptr references 'a' ptr = &a; printf("%x\n",ptr); //increment pointer address by 1 ptr++; printf("%x\n",ptr); return 0; }
Let's discuss
Integer variable will take 4 bytes in memory to store the value.
If we add 1 to integer pointer address, it will point to the next integer starting address which is current base address + sizeof(int).
In this case output will be 1024 + 4 => 1028.
Pictorial Explanation
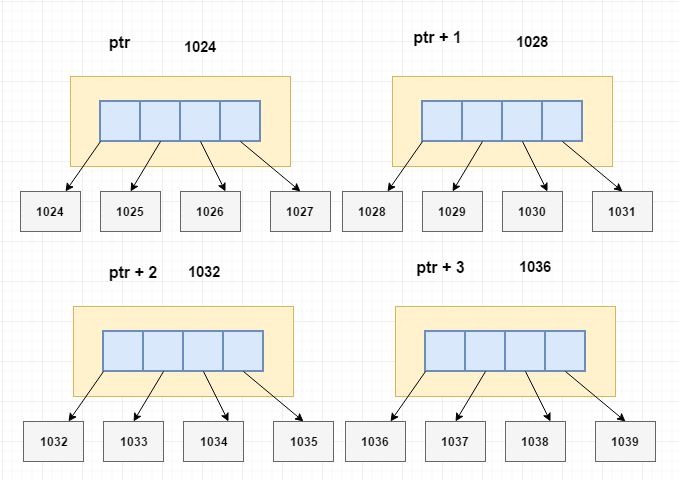