Integer pointer
Integer pointer only stores the address of an integer variable.
Syntax
int *pointer_name;
How to assign a variable address to the pointer?
This is also called referencing which means assigning the address of an existing variable to a pointer.
To reference a variable to the pointer, we should use & operator.
int a = 10; int *ptr; ptr = &a; //here ptr references 'a'
Where,
a is an integer variable which holds the value 10.
ptr is a pointer to a variable with type integer which holds the address of variable 'a'.
Like below,
Pictorial Explanation
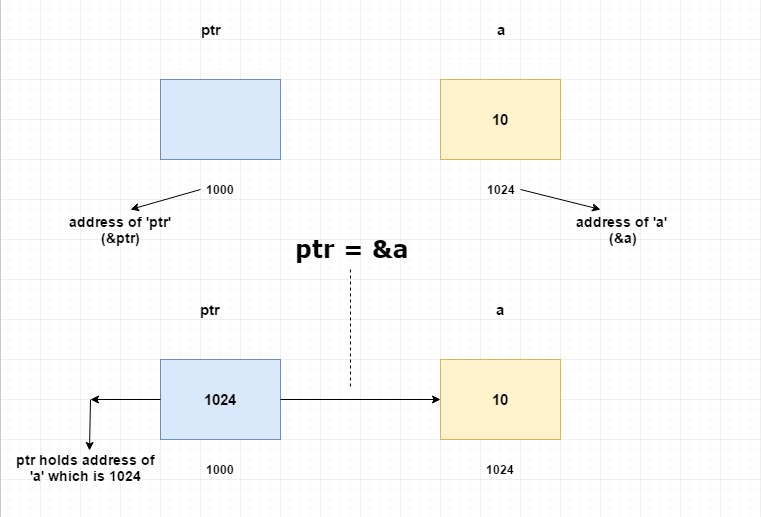
How to access the value stored at the pointer?
Using * (asterisk) operator, we can access the value stored at the pointer.
It is also called the dereference operator which will give the value stored at the pointer.
*ptr will give the value stored at the pointer.
Example
printf("value stored at ptr = %d",*ptr);
*ptr => *(1024) => value at memory address 1024 is 10.
Animated Tutorial
Program
Example
#include<stdio.h> int main() { int a = 10; int *ptr; ptr = &a; printf("value of a = %d\n",a); printf("value stored at ptr = %d\n",*ptr); printf("Address of a = %x\n",&a); printf("ptr points to the address = %x\n",ptr); printf("Address of ptr = %x\n",&ptr); return 0; }
Output
From the above diagram.
value of a = 10 value stored at ptr = 10 Address of a = 1024 ptr points to the address = 1024 Address of ptr = 1000