malloc function
Using malloc function, we can create memory dynamically at runtime.
malloc is declared in <stdlib.h> i.e. standard library header file.
To use malloc function in our program, we have to include <stdlib.h> header file.
syntax of malloc
malloc(size in bytes);
Example
char *ptr; ptr = malloc(10);
malloc will take only one argument.
where,
ptr is a pointer. It can be any type.
malloc - used to create dynamic memory
10 - It will allocate 10 bytes of memory.
How does malloc work?
malloc will create the dynamic memory with given size and returns the base address to the pointer.
If malloc unable to create the dynamic memory, it will return NULL.
So, it is mandatory to check the pointer before using it.
Example
//allocating memory for 5 integers using malloc int *ptr = malloc(5*sizeof(int));
Pictorial Explanation
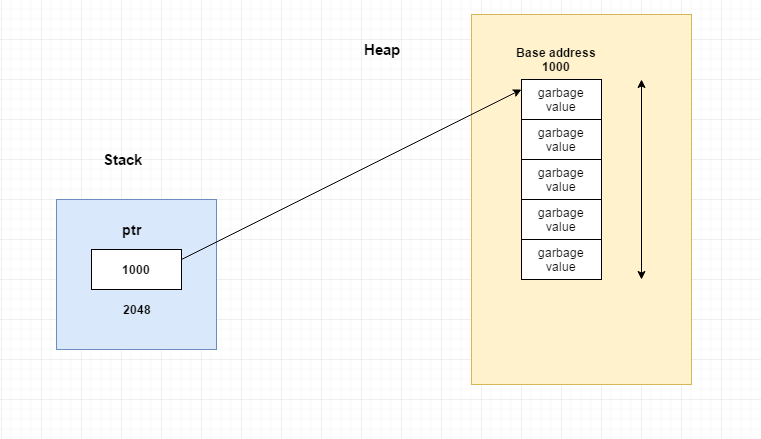
malloc will create 20 ( 5 * 4 ) bytes of memory and return the base address to pointer variable ptr on success.
Initialization
malloc doesn't initialize the memory area which is created dynamically.
So, the memory area will have garbage values.
Animated Tutorial
Let's create a dynamic memory using malloc
Example
/* *Dynamic memory creation using malloc *Language: C */ #include<stdio.h> //To use malloc function in our program #include<stdlib.h> int main() { int *ptr; //allocating memory for 1 integer ptr = malloc(sizeof(int)); if(ptr != NULL) printf("Memory created successfully\n"); return 0; }
Let's get size input from the user and allocate the dynamic memory using malloc.
Example
/* *Dynamic memory creation using malloc *Language : C */ #include<stdio.h> //To use malloc function in our program #include<stdlib.h> int main() { int *ptr,size,i; scanf("%d",&size); ptr = malloc(size * sizeof(int)); if(ptr != NULL) { //let's get input from user and print it printf("Enter numbers\n"); for(i = 0; i < size; i++) scanf("%d",ptr+i); //printing values printf("The numbers are\n"); for(i = 0; i < size; i++) printf("%d\n",*(ptr+i)); // *(ptr+i) is as same as ptr[i] } return 0; }