Pointer argument to function
We can also pass the pointer variable to function.
In other words, we can pass the address of a variable to the function instead of variable value.
How to declare a function which accepts a pointer as an argument?
If a function wants to accept an address of an integer variable then the function declaration will be,
return_type function_name(int*);
* indicates that the argument is an address of a variable not the value of a variable.
Similarly, If a function wants to accept an address of two integer variable then the function declaration will be,
return_type function_name(int*,int*);
What will happen to the argument?
Here, we are passing the address of a variable.
So, if we change the argument value in the function, it will modify the actual value of a variable.
Example
#include<stdio.h> void setToZero(int *a) { *a = 0; } int main() { int a = 10; printf("Before calling the function a = %d\n",a); setToZero(&a); printf("After calling the function a = %d\n",a); return 0; }
Output
Before calling the function a = 10
After calling the function a = 0
Visual Representation
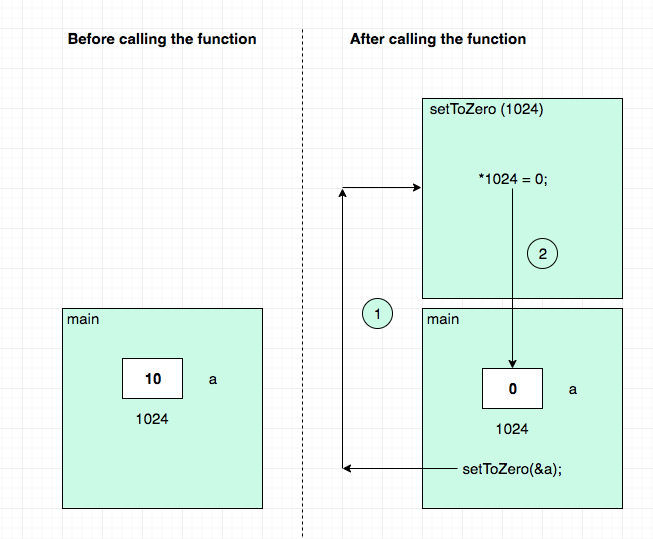
step 1. Address of a variable a 1024 is passed to function setToZero().
step 2. *a = 0 will modify the original value of a. So, a value in main function will be 0 after the function execution.