Pointer to an array
We can also point the whole array using pointers.
Using the array pointer, we can easily manipulate the multi-dimensional array.
Example
int arr[5] = {10, 20, 30, 40, 50}; int (*ptr)[5]; ptr = &arr;
Where, ptr points the entire array.
Dereferencing the array pointer
Since ptr is an array pointer,
*ptr will be again an address which is the address of the first element in the array.
**ptr will be the value stored at the address.
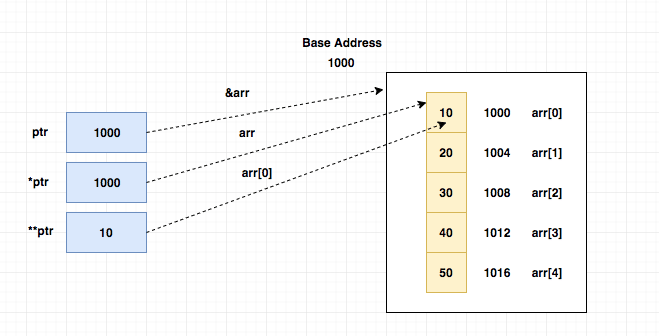
Example
/* * Pointer to an array example */ #include<stdio.h> int main() { int arr[5]={10, 20, 30, 40, 50}; int (*ptr)[5]; //pointer to an array of 5 integers ptr = &arr; //ptr references the whole array printf("Address of the array = %p\n",ptr); printf("Address of the first element in the array = %p\n", *ptr); printf("Value of the first element = %d\n",**ptr); return 0; }
ptr+1 vs *ptr+1
ptr + 1 | *ptr + 1 |
---|---|
ptr is a pointer to an entire array. So, if we move ptr by 1 position it will point the next block of 5 elements. |
*ptr is a pointer to the first element of the array.So, if we move *ptr by 1 position it will point the second element. |
If the array base address is 1000,ptr+1 will be 1000 + (5 * 4) which is 1020 |
If the array base address is 1000, *ptr+1 will be 1000 + 4 which is 1004 |
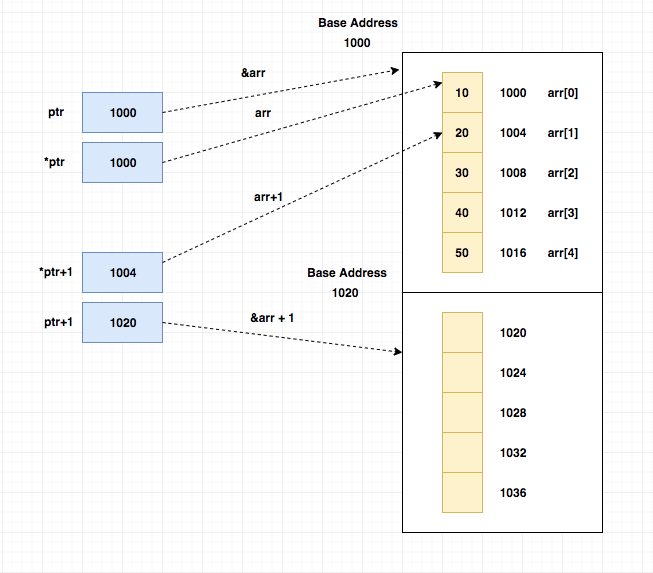
Example
/* * Pointer to an array */ #include<stdio.h> int main() { int arr[5]={10, 20, 30, 40, 50}; int (*ptr)[5]; //pointer to an array of 5 integers ptr = &arr; //ptr references the whole array /* *ptr is a pointer to the whole array *ptr+1 will point the next block of 5 elements */ printf("ptr = %p \t ptr+1 = %p\n",ptr,ptr+1); /* *ptr is a pointer to the first element in the array *ptr+1 will point the next element in the array */ printf("*ptr = %p \t *ptr+1 = %p\n",*ptr,*ptr+1); return 0; }