Pointer to pointer in c
We can also store the address of another pointer in c.
This tutorial explains pointer to pointer concept with examples.
Example
int a = 10; int *ptr = &a;
Where, ptr is a pointer which is holding the address of variable a.
If we need to hold the address of ptr, what will be the solution?
Pointer to Pointer.
Declaration of pointer to pointer (double pointer)
int a = 10; int *ptr = &a; int **dptr = &ptr;
We need to use ** to declare the pointer to pointer(double pointer) variable.
** declaration indicates that I am a pointer but I am not pointing to a variable rather pointing to the address of another pointer.
Visual Representation of the above code
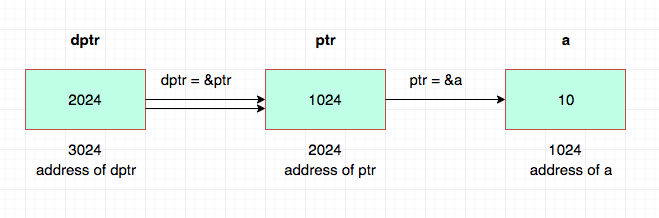
Referencing and dereferencing a double pointer
i)Referencing
Assigning an address to the pointer.
ii)Dereferencing
Accessing the value stored at the pointer.
Example
Example
/* * Program : Pointer to Pointer * Language : C */ #include<stdio.h> int main() { int a = 10; int *ptr = &a; //ptr references a int **dptr = &ptr; //dptr references ptr printf("Address of a = %p\n",&a); printf("ptr is pointing to the address = %p\n",ptr); printf("dptr is pointing to the address = %p\n",dptr); printf("Value of a = %d\n",a); printf("*ptr = %d\n",*ptr); printf("**dptr = %d\n",**dptr); return 0; }
*ptr -> *1024 -> value stored at the memory 1024 is 10 ->10
**dptr -> *(*2024)-> value stored at the memory address 2024 is 1024 -> *1024->value stored at the memory address 1024 is 10 -> 10
Find the output
Using the above diagram, can you find the output of the program?
Address of a = ____________
ptr is pointing to the address = ____________
dptr is pointing to the address = ___________
Value of a = ___________
*ptr = ___________
**dptr = ____________