realloc in c
Use of realloc function
Using realloc function, we can resize the memory area which is already created by malloc or calloc.
It's is also declared in stdlib.h library.
Limitation
If the memory area is not created dynamically using malloc or calloc, then the behavior of the realloc function is undefined.
realloc syntax
realloc(ptr, new size);
Where,
realloc - used to resize the memory area which is pointed by ptr.
ptr - the name of the pointer variable which needs to be resized.
new size - the new size of the memory area. It can be smaller or bigger than the actual size.
return value of realloc
If realloc request success, it will return a pointer to the newly allocated memory.
Otherwise, it will return NULL.
Example
Changing size from 100 bytes to 1000 bytes using realloc.
char *ptr; ptr = malloc(100); ptr = realloc(ptr,1000);
How realloc works?
1.realloc will act as malloc if the pointer variable is NULL.
Example
#include<stdio.h> //To use realloc in our program #include<stdlib.h> int main() { char *ptr; ptr = NULL; /* *since the ptr is NULL, *it will act like malloc function */ ptr = realloc(ptr,10); if(ptr != NULL) printf("Memory created successfully\n"); return 0; }
realloc smaller size
2.If the given size is smaller than the actual, it will simply reduce the memory size.
Example
#include<stdio.h> //To use realloc in our program #include<stdlib.h> int main() { int *ptr; //allocating memory for 10 integers ptr = malloc(10 * sizeof(int)); //realloc memory size to store only 5 integers ptr = realloc(ptr, 5 * sizeof(int)); return 0; }
3.If the given size is bigger than the actual, it will check whether it can expand the already available memory.
If it is possible it will simply resize the memory.
Example
#include<stdio.h> //To use realloc in our program #include<stdlib.h> int main() { int *ptr,i; //allocating memory for only 1 integer ptr = malloc(sizeof(int)); ptr[0] = 1; //realloc memory size to store 3 integers ptr = realloc(ptr, 3 * sizeof(int)); ptr[1] = 2; ptr[2] = 3; //printing values for(i = 0; i < 3; i++) printf("%d\n",ptr[i]); return 0; }
Otherwise, realloc will create the new block of memory with the larger size and copy the old data to that newly allocated memory and than it will deallocate the old memory area.
For example assume, we have allocated memory for 2 integers.We are going to increase the memory size to store 4 integers.
If realloc unable to expand the memory size, it will do something like below,
Pictorial Explanation
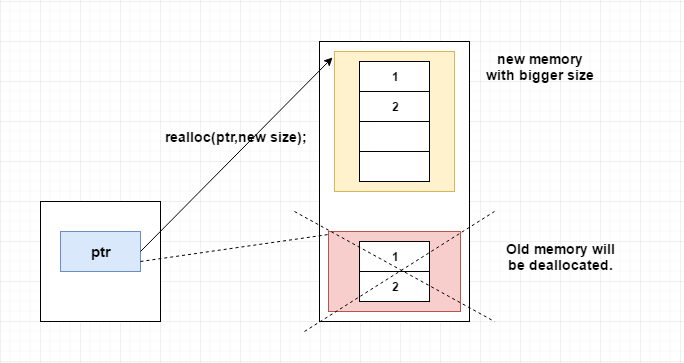
Animated Tutorial
Sample Program
Example
#include<stdio.h> //To use realloc in our program #include<stdlib.h> int main() { int *ptr,size,i; /* * Let's create memory for 2 integers * size = 2 */ size = 2; ptr = malloc(size * sizeof(int)); // *(ptr + i) === ptr[i] *(ptr + 0) = 1; *(ptr + 1) = 2; //printing elements for(i = 0; i < size; i++) printf("%d\n",ptr[i]); /* * Let's change the size to store 3 more integers * size = 5 */ size = 5; ptr = realloc(ptr, size * sizeof(int)); *(ptr + 2) = 3; *(ptr + 3) = 4; *(ptr + 4) = 5; for(i = 0; i < size; i++) printf("%d\n",ptr[i]); return 0; }