String Manipulation
As we discussed earlier, string is a sequence of characters terminated by null character ‘\0’.
In this tutorial, we will learn how to play with the characters in the string.
Let’s take a sample string.
Example
char word[6]=”HELLO”;
Pictorial Explanation
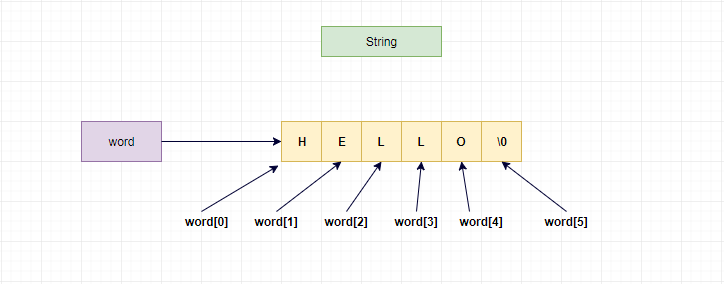
String manipulation in C
We can access each character in the string using index value like array.
String index also starts with 0.
In general,
Nth character of the string can be accessed by using index N-1.
Because string index starts with 0 Not 1.
Example
To access 1st character of word, we should use index 0.
Similarly,
To access 4th character of word, we should use index 3.
Program
Example
/* string manipulation */ #include<stdio.h> int main() { char word[6]="HELLO"; printf("%s\n",word); //printing unchanged string word[4] = '*'; //changing word index 4 as '*'. printf("%s\n",word); //It will print "HELL*" word[1] = '#'; //changing word index 1 as '#'. printf("%s\n",word); //It will print "H#LL*" return 0; }
Pictorial Explanation
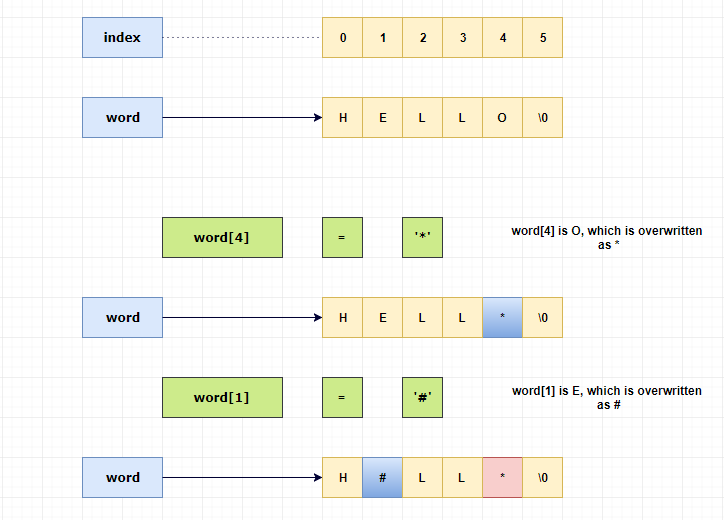