Structure within structure
We can have a structure variable as a member of another structure.
Example
Let's create a structure to store the coordinates (x,y).
Example
struct coordinate { int x; int y; };
Using two coordinates, we can draw a line { (x1,y1), (x2,y2) }.
To achieve that, we can create another structure and store two coordinates(struct coordinates variable).
Like below,
Example
struct line { struct coordinate c1; struct coordinate c2; };
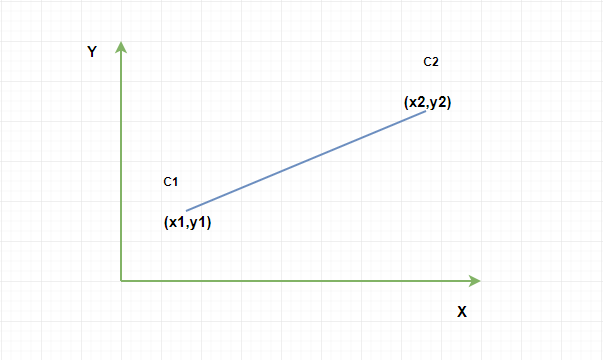
Accessing structure members
Using below method, we can access the structure members.
(outer structure variable).(inner structure variable).members
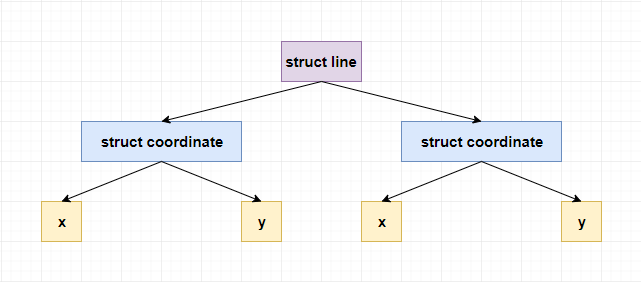
In our case,
Example
To access X struct line variable. struct coordinate variable.x To access Y struct line variable. struct coordinate variable.y
Let's create a variable for struct line.
struct line l;
Example
To access x and y of coordinate 1 (c1),
l.c1.x l.c1.y
Similarly for coordinate 2 (c2),
l.c2.x l.c2.y
Let's find the distance between the two coordinates
Distance = √ (x2-x1)2 + (y2-y1)2
Example
#include<stdio.h> //To use sqrt and pow function #include<math.h> int main() { float distance; //To store x and y struct coordinate { int x; int y; }; //To store two coordinates struct line { struct coordinate c1; struct coordinate c2; }; //structure line variable struct line l; //get coordinate 1 printf("Enter c1(x and y)\n"); scanf("%d%d",&l.c1.x,&l.c1.y); //get coordinate 2 printf("Enter c2(x and y)\n"); scanf("%d%d",&l.c2.x,&l.c2.y); /* * *calculate distance * /----------------------- * __/(x2-x1)^2 + (y2-y1)^2 * * #include<math.h> * sqrt(4) = 2 (Square root) * pow(x,2) = x * x (Power) * */ distance = sqrt(pow((l.c2.x - l.c1.x),2) + pow((l.c2.y - l.c1.y),2)); printf("Distance = %f\n",distance); return 0; }