Linear Search | Sequential Search
Assume that, I am going to give you a book which has unordered page numbers. And it has 100 pages.
Like,
First page marked as number 56.
Second page marked as number 89.
And so on.
Now, what will you do if I ask you to find the page number 50?
Since the page numbers are unordered, we don’t have any choice other than searching it page by page.
Example
Take first page. If it is page number 50. We are done.
Or else goto the next page and do the same process until we find the page number 50.
If we run out of page, we can conclude that page number 50 is not in the book.
This is called as Linear Search or Sequential Search.
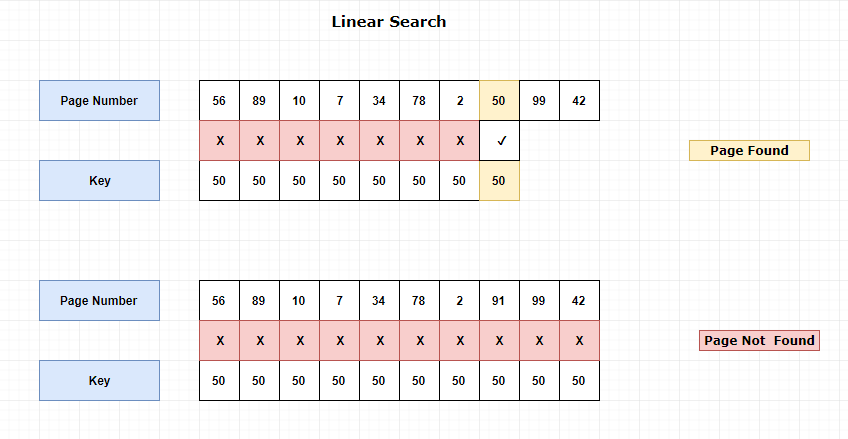
Linear Search Program in C
/* * Program: Linear Search * Language: C */ #include<stdio.h> #define SIZE 5 //size of the array /* * It will return 1, if search found * Otherwise it will return 0 */ int LinearSearch(int arr[], int size, int key) { int i; //if any match, return 1 for(i = 0; i < size; i++) if(arr[i] == key) return 1; /* * Out of the loop * Which means Key not present in the array, * so return 0. */ return 0; } int main() { //array of elements int page_number[SIZE] = {86,91,34,50,0}; //key to search int key = 50; if(LinearSearch(page_number, SIZE, key) == 1) printf("Search Found"); else printf("Search Not Found"); return 0; }