C program to find sum of array elements
Write a program to find the sum of all elements in the given array.
Example
Input
array size = 5
Elements = { 10, 2, 5, 0, 30}
Output
47
Input
array size = 4
Elements = {12, -1, 0, 8}
Output
19
Procedure
1.Declare a variable to store the sum. Say int sum;.
2.We should initialize the sum variable to 0.i.e. sum = 0;
3.Loop through all the elements in the array and add them to variable sum.
4.Print the sum.
If we don't initialize sum as 0, sum will take some garbage value
All the elements will be added to the garbage value.
And it will result the garbage output.
Sum of the array
Example
#include<stdio.h> int main() { //let's assume the maximum array size as 100. //initialize sum as 0. Otherwise, it will take some garbage value. int arr[100], size, i, sum = 0; //Get size input from user printf("Enter array size\n"); scanf("%d",&size); //Get all elements using for loop and store it in array printf("Enter array elements\n"); for(i = 0; i < size; i++) scanf("%d",&arr[i]); //add all elements to the variable sum. for(i = 0; i < size; i++) sum = sum + arr[i]; // same as sum += arr[i]; //print the result printf("Sum of the array = %d\n",sum); return 0; }
Step by Step Execution Result
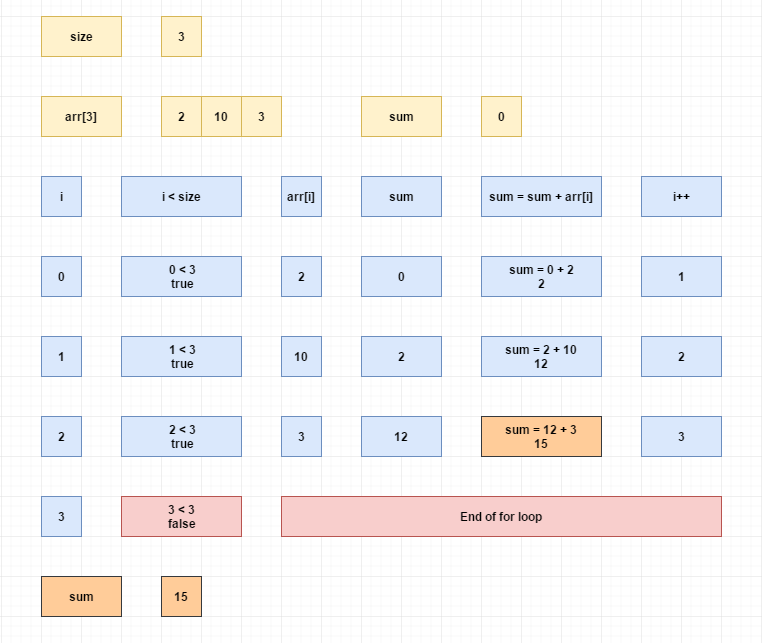