Odd or Even - bitwise solution
We can solve the odd or even problem more efficiently using bitwise operators.
Let’s represent numbers in binary format and we will find some logic to solve this problem.
Pictorial Explanation
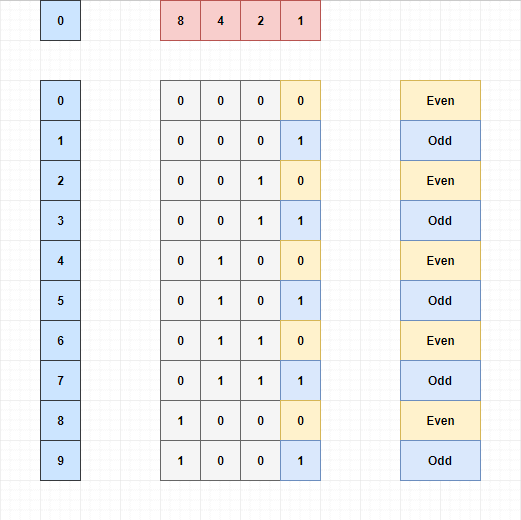
Analysis
If we observe the above diagram, we can conclude that
LSB (least significant bit) of Odd number is 1
LSB of even number is 0.
Now, all we need to do is, just check the LSB of a number. If it is 1, it is Odd number. If it is 0, the number is even.
Using Bit-wise AND operator, we can check whether the LSB is 0 or 1.
Example
Let’s take number 10. We have to check whether the LSB is 1 or 0.
To check that,
We need to create a bit mask with LSB bit as 1 and all bits are 0. Because we are going to check only the LSB bit.
So, Bit Mask will be,
(00000000000000000000000000000001) 2 which 110
Now do Number & Bit Mask,
if result is 1, it is an Odd number.
else, it is an even number.
Solution
Get a number from the user
If number & 1 is 0
    Even
Else
    Odd
Pictorial Explanation
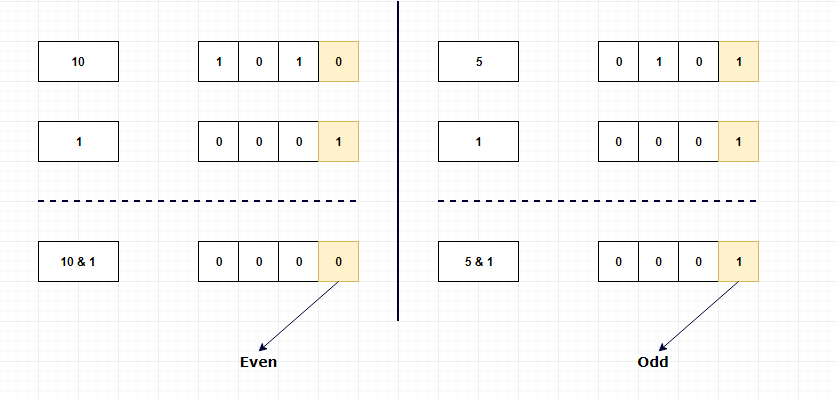
Program
Example
#include<stdio.h> int main() { int number; scanf("%d",&number); if((number & 1) == 0) printf("Even"); else printf("Odd"); return 0; }