Factorial of a number
Factorial(n) = number of ways of arranging a set of n objects.
Factorial of empty set i.e 0
n = 0
empty set = {}
There is only one way to arrange the empty set.
{}
so, 0! = 1
Factorial of 1
n = 1
set ={1}
We can arrange it in one way
{1}
so, 1! = 1
Factorial of 2
n = 2
set = {1,2}
we can arrage it in two ways like below,
{1,2}
{2,1}
so 2! = 2
Factorial of 3
n = 3
set = {1,2,3}
we can arrange it in 6 ways like below,
{1,2,3}
{1,3,2}
{2,1,3}
{2,3,1}
{3,1,2}
{3,2,1}
so 3! = 6
Pictorial Explanation
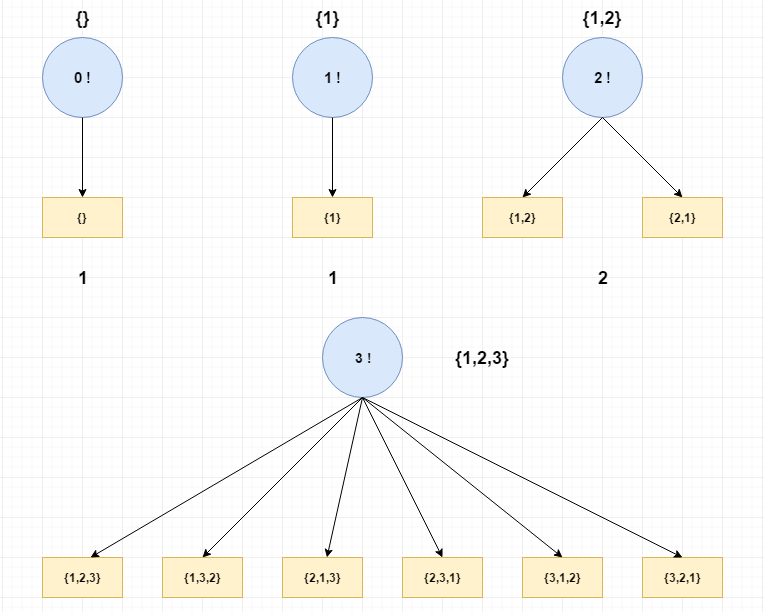
In general,
n factorial =
n >= 0     n! = n * n -1 * n-2 * n-3 ...* 1
n < 0       Not defined
Example
3! = 3 * 2 * 1
6
5! = 5 * 4 * 3 * 2 * 1
120
Let's write a code
Example
#include<stdio.h> int main() { //set factorial variable as 1. //if we set that as 0, end result will be zero int n,i,factorial = 1; //get input from user scanf("%d",&n); if(n < 0) printf("Enter a non-negative number\n"); else { /*if n = 0 or n = 1, the loop will not be executed *initial value will be printed that is 1. (factorial = 1) */ //if n = 5, ==> 2 * 3 * 4 * 5 //if n = 3, ==> 2 * 3 for(i = 2; i <= n; i++) factorial = factorial * i; printf("%d! = %d",n,factorial); } return 0; }