Reverse a number
Previous article, we have learnt how to split the digits from a number.
Now,
Get a positive number from user and we have to print the reversed number.
Example
Case 1
Input
12
Output
21
Case2
Input
1234
Output
4321
Logic
set ans = 0
till num > 0
do
mod = num % 10              // split the last digit
ans = ans * 10 + mod     // multiply ans with 10 and add the last digit
num = num / 10              // divide number by 10
Pictorial Explanation
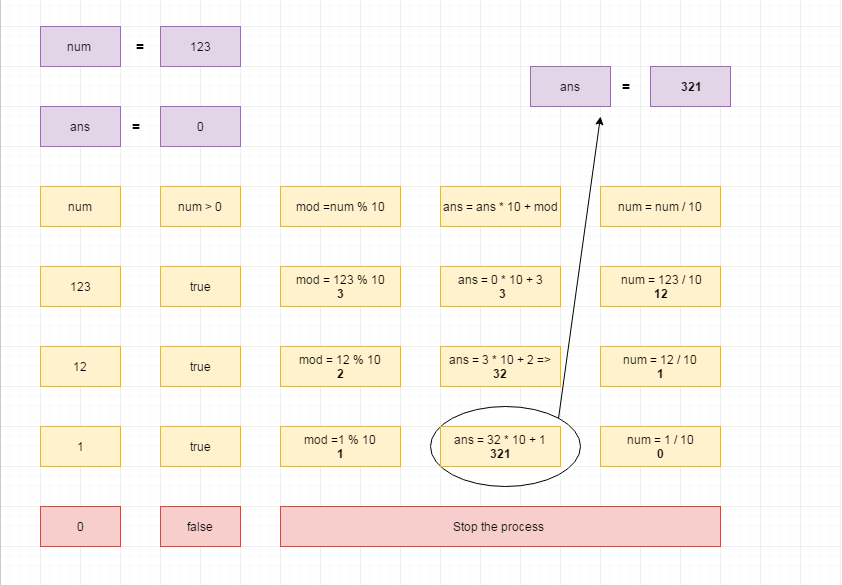
Program
Example
#include<stdio.h> int main() { int num, ans = 0; //get input from user scanf("%d",&num); //do till num > 0 while(num > 0) { //split the last digit int mod = num % 10; //multiply ans with 10 and add the splitted digit ans = ans * 10 + mod; //divide num by 10 num = num / 10; } //print the reversed number printf("Reversed Number is %d\n",ans); return 0; }