An array is a linear data structure
An array is a collection of variables in the same datatype.
we can’t group different data types in the array. Like, a combination of integer and char, char and float etc.
Hence array is called as the homogeneous data type.
Example
int arr[5]={10,20,30,40,50};
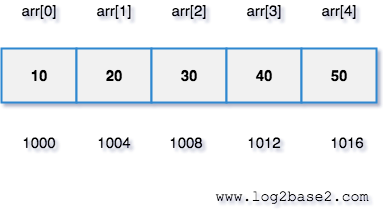
Array Index
Using index value, we can directly access the desired element in the array.
Array index starts from 0, not 1.
To access the 1st element, we can directly use index 0. i.e age[0]
To access the 5th element, we can directly use index 4. i.e age[4]
We can manipulate the Nth element by using the index N - 1. {Where N > 0}
In general, an array of size N will have elements from index 0 to N-1.
Accessing array elements using index
/* * Accessing array elements using index */ #include<stdio.h> int main() { int arr[5] = {10, 20, 30, 40, 50}; //printing 3rd element which is index 2 printf("3rd element = %d\n", arr[2]); //printing 5th element which is index 4 printf("5th element = %d\n", arr[4]); return 0; }
Time complexity analysis
Using the index value, we can access the array elements in constant time.
So the time complexity is O(1) for accessing an element in the array.