inserting a node at the end of a linked list
The new node will be added at the end of the linked list.
Example
Input
Linked List : 10 20 30 40 NULL.
50
Output
Linked List : 10 20 30 40 50 NULL.
Input
Linked List : NULL
10
Output
Linked List : 10 NULL
Algorithm
1. Declare head pointer and make it as NULL.
2. Create a new node with the given data. And make the new node => next as NULL.
    (Because the new node is going to be the last node.)
3. If the head node is NULL (Empty Linked List),
         make the new node as the head.
4. If the head node is not null, (Linked list already has some elements),
         find the last node.
         make the last node => next as the new node.
1. Declare head pointer and make it as NULL.
struct node { int data; struct node *next; }; struct node *head = NULL;
2. Create a new node
void addLast(struct node **head, int val) { //create a new node struct node *newNode = malloc(sizeof(struct node)); newNode->data = val; newNode->next = NULL; }
3. If the head node is NULL, make the new node as head
void addLast(struct node **head, int val) { //create a new node struct node *newNode = malloc(sizeof(struct node)); newNode->data = val; newNode->next = NULL;//if head is NULL, it is an empty list if(*head == NULL) *head = newNode;}
Visual Representation
Let's insert data 10.
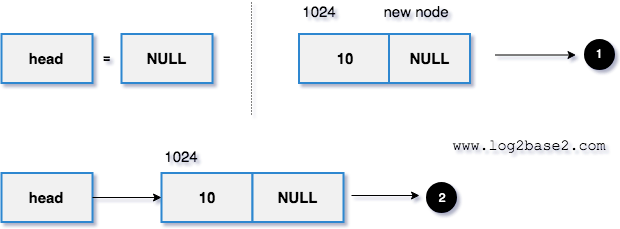
The head is NULL initially.
1. The new node with data as 10 and reference is NULL (address 1024).
2. Since it is the first node, make the head node points to the newly allocated node.
4. Otherwise, find the last node and set last node => new node
The last node of a linked list has the reference pointer as NULL. i.e. node=>next = NULL.
To find the last node, we have to iterate the linked till the node=>next != NULL.
Pseudocode
while(node->next != NULL) { node = node->next; }
After that, we have to make the last node-> next as the new node. i.e. last node->next = new node;
void addLast(struct node **head, int val) { //create a new node struct node *newNode = malloc(sizeof(struct node)); newNode->data = val; newNode->next = NULL; //if head is NULL, it is an empty list if(*head == NULL) *head = newNode; //Otherwise, find the last node and add the newNode else { struct node *lastNode = *head;//last node's next address will be NULL. while(lastNode->next != NULL) { lastNode = lastNode->next; } //add the newNode at the end of the linked list lastNode->next = newNode;} }
Visual Representation
Let's insert data 20.
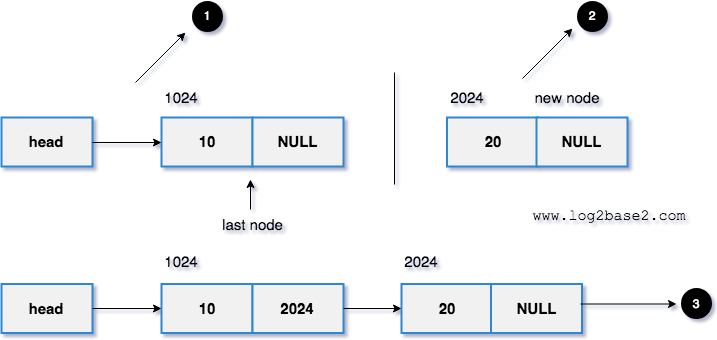
1. The head points to the memory address 1024 and it is the last node.
2. The new node with data as 20 and reference is NULL (address 2024).
set last node =>next = new node. The new node added at the end of the linked list.
3. Finally, the new linked list.
Let's insert data 30.
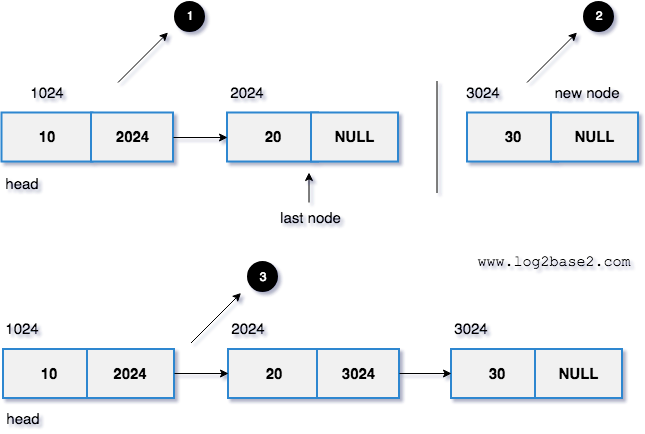
1. The head points to the memory address 1024 and it has two nodes.
2. The new node with data as 30 and reference is NULL (address 3024).
Find the last node using the loop and make the last node points to the newly allocated node.
In our case, the last node is 20 (address 2024). So, last->next = 30 (address 3024).
3. Finally, the new linked list.
Implementation of inserting a node at the end of a linked list
Example
#include<stdio.h> #include<stdlib.h> struct node { int data; struct node *next; };void addLast(struct node **head, int val) { //create a new node struct node *newNode = malloc(sizeof(struct node)); newNode->data = val; newNode->next = NULL; //if head is NULL, it is an empty list if(*head == NULL) *head = newNode; //Otherwise, find the last node and add the newNode else { struct node *lastNode = *head; //last node's next address will be NULL. while(lastNode->next != NULL) { lastNode = lastNode->next; } //add the newNode at the end of the linked list lastNode->next = newNode; } }void printList(struct node *head) { struct node *temp = head; //iterate the entire linked list and print the data while(temp != NULL) { printf("%d->", temp->data); temp = temp->next; } printf("NULL\n"); } int main() { struct node *head = NULL; addLast(&head,10); addLast(&head,20); addLast(&head,30); printList(head); return 0; }