Assignment Operator in Python
Using assignment operators, we can assign value to the variables.
Equality sign (=) is used as an assignment operator in Python.
Example
var = 5
Here, an integer object with value 5 has assigned to the variable var.
Both 'a' and 'b' will point the same integer object which has the value 5. So, both a and b will hold value 5.
Basically, the value of right-side operand will be assigned to the left side operand.
Pictorial Explanation
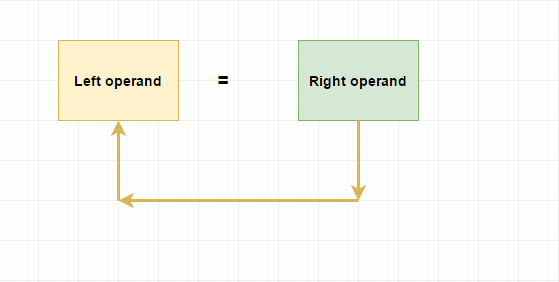
Compound assignment operators in python
Operator | Meaning | Example (a = 10 , b = 5) |
---|---|---|
+= | L=L+R add left and right operand and assign result in left |
a+=b;same as a=a+b after execution a will hold 15 |
-= | L=L-R subtract right operand from left operand and assign result in left |
a-=b;same as a=a-b after execution a will hold 5 |
*= | L=L*R multiply both right and left operand and store result in left |
a*=b;same as a=a*b after execution a will hold 50 |
/= | L=L/R divides left operand by right operand and store result in left |
a/=b;same as a=a/b after execution a will hold 2 |
%= | L=L%R After left and right operand division, the remainder will be stored in left |
a%=b;same as a=a%b after execution a will hold 0 |
**= | L=L**R L will hold the value of L R. i.e pow(L,R). |
a**=b;same as a=a**b after execution a will hold 100000 |
//= | L=L//R L will have the floor division value of L // R. |
a//=b;same as a=a**b after execution a will hold 2. |