Shell script to print sum of all digit
Let's write a shell script to print the sum of all digits in a given number.
Algorithm
1. Get a number
2. Split each digit from the number using modulo operator.
3. Calculate the sum
4. Print the result.
Sum of all digits - Shell Script
echo "Enter a number" read num sum=0 while [ $num -gt 0 ] do mod=$((num % 10)) #It will split each digits sum=$((sum + mod)) #Add each digit to sum num=$((num / 10)) #divide num by 10. done echo $sum
Shell script to print sum of all digit using expr
#sum of all digits - shell script echo "Enter a number" read num sum=0 while [ $num -gt 0 ] do mod=`expr $num % 10` #It will split each digits sum=`expr $sum + $mod` #Add each digit to sum num=`expr $num / 10` #divide num by 10. done echo $sum
Visual Representation
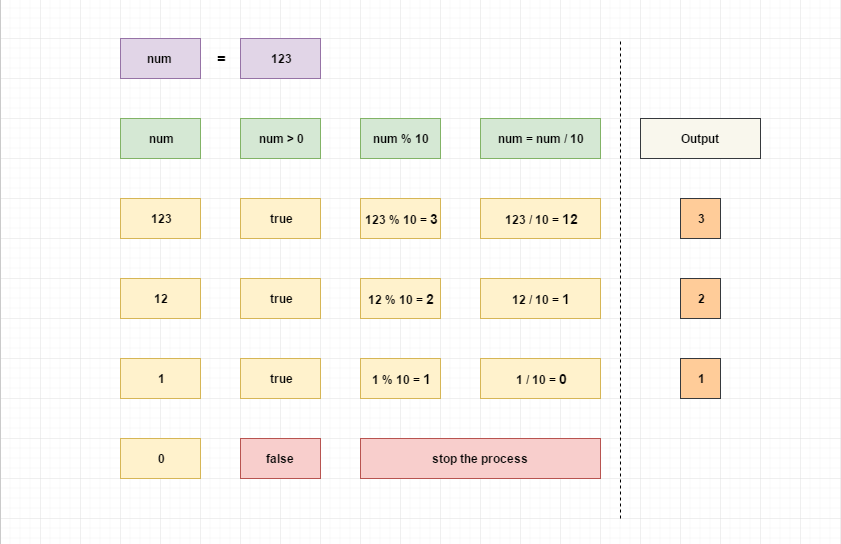
In our algorithm, we just added each digit to the sum.
Output
Enter a number
100
1
Enter a number
786
21
Useful Resources
To learn more shell script examples, you can visit the link